1. Introduction #
Modular code construction is a way to reduce maintenance of your RPA processes. The idea is to build a large process that has modules that can be reused across multiple smaller processes. Section 2 is documentation of just such a process, built in Power Automate Desktop , where you journalize digital forms for an ESDH system. Since the code is largely the same whether you journalize one form or multiple ones, it is an ideal process to make it modular.
Name | Note | Link |
Coding – Structuring | The theory from section 3 here is also used in this review. | https://www.rpahelp.org/docs/kodestruktur/#6-toc-title |
KMD SAP | Section 3 herein is another example of a modular process. | https://www.rpahelp.org/docs/kmd-sap/#6-toc-title |
1.1 What is a module? #
A module is generic code that is reusable in multiple contexts. If we look at the code for 30.30 – Journalize files below, it looks like this. In short, with an API call, an attempt is made to journalize a list of files. If this is not successful, there is built-in debugging that handles the error itself. In addition, the module returns a DocumentIdentifier , a unique ID in the ESDH system for the specific document, as well as a JSON that may contain several variables that can be used subsequently.
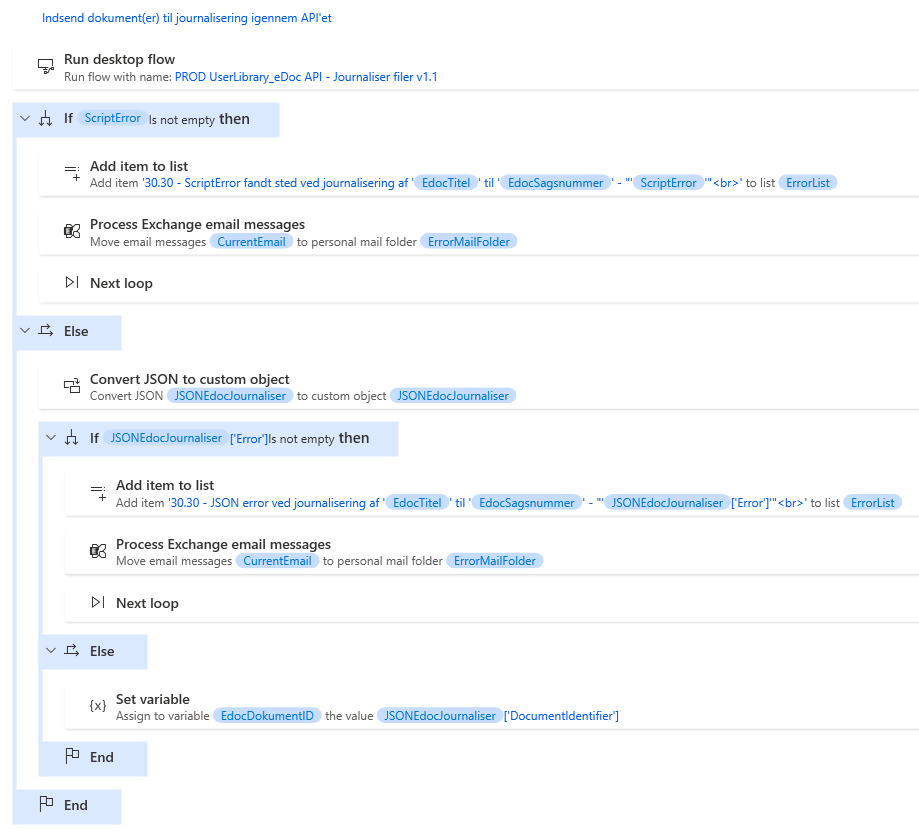
1.2 How is a module used? #
Continuing with the example, a workflow in a modular RPA process can look like this. Before we run the modules, the variables that we want to use in this are set. Subsequently, we can use what the module sends back further in the workflow. See the last Region , where variables are set based on the JSON that comes after Run subflow 30.10 .
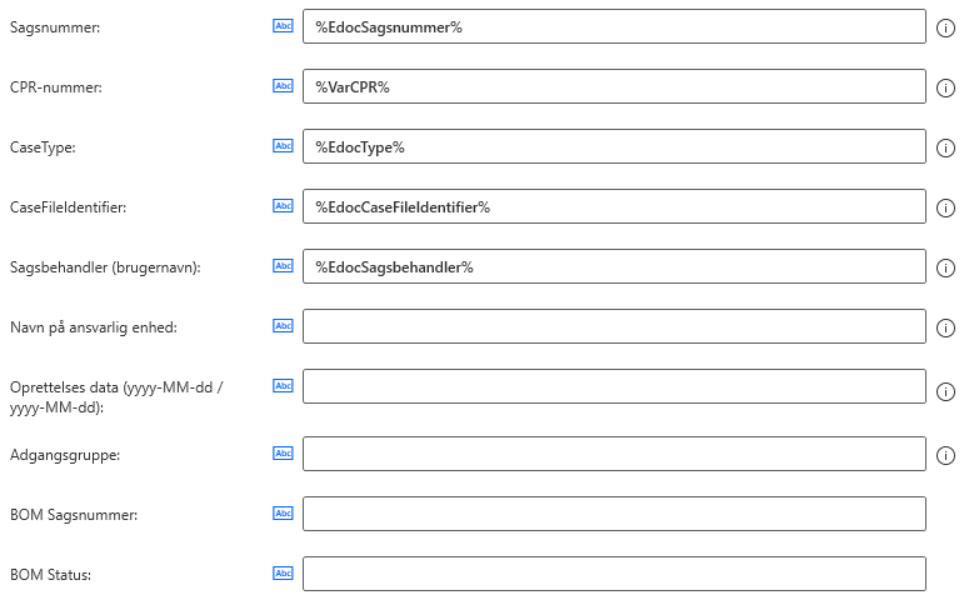
2. XFlow for eDoc – Journalize forms ALL #
2.1 Description #
The code itself is designed to work in the bot users’ inbox, where it reviews emails from XFlow, and journalizes forms for eDoc cases. This means that as the need arises, you can continuously set up new cases for either bot users in 10.10 or forms in 20.10.
List of forms that the robot handles:
Subject area | Blank | Comment |
DOVE | Exemption from German | |
WISE | Diet and Transportation | Takes care of all 6x forms ( Resident Complaints Board, Grants Board, Children and Youth Committee, Disability Council, Fence Inspection, Rent Board ) |
2.2 Code description #
Brief overview of the variables used for the eDoc API and what they do:
Name | Description |
BoolEdocDocument | Used to activate an else-if so that the robot extracts the EdocDokumentID from the JSON |
BoolEdocProject | Used to activate an else-if so that the robot extracts the EdocProjectID from the JSON |
EdocCaseFileIdentifier | A case’s unique ID in the backend |
EdocDocumentDate | Required when journaling, as a rule, date dd-mm-yyyy. Can be exceeded if necessary, as it is reset between each loop. |
EdocDocumentID | A document’s unique ID in the backend |
EdocDocumentNo | A document’s number towards the user interface |
EdocCategory | The ID of the category to be used, for list see: R:\SCRIPTS\PowerShell\eDoc\GetItemList\edoc\CaseCategory.txt |
EdocCreateCase | Used in a Switch to determine what to create |
EdocProjectID | A project’s unique ID in the backend |
EdocProjectNo. | A project’s number towards the user interface |
EdocCaseworker | The initials of a caseworker |
EdocCase number | A case number against the user interface |
EdocTemplate | The template to be used for creation |
EdocSearchFor | Used in a Switch to determine what to search for |
EdocTitle | A title in eDoc either for searching or creation |
EdocType | eDoc case type: Deleted case Administrative case Civil case Property case PPR – Case |
JSONEdocJournalize JSONEdocCreate JSONEdocSearch | The respective JSON files that are loaded after each API call. As a rule, they only return an EdocCaseFileIdentifier , EdocProjektID or EdocDokumentID . The rest you have to do in your code if you need to extract further. |
The code is divided into the following subflows, in which there are comments with #TODO in the code if you actively need to make changes:
Main : This is the main function that orchestrates the entire process. From here, other functions are called in sequence to handle different parts of the workflow.
00.10 Initialize : Prepares the environment by setting up necessary variables, directories, and logging details. This includes variables for debugging and logging to SQL, which must be overridden for new use.
99.99 Exit : Handles the cleanup and logging at the end of the process, including sending error reports if necessary.
Global Error Handler : Provides a mechanism to catch and log errors that occur during the execution of the automation that would stop the process from running completely. This ensures that any issues are detected and an error message is sent to the developer.
00.20 – MonthNumber to MonthName: Converts the month of the run date to the month name in the “MonthName” variable. Intended if you need to name cases / documents with the current month.
10.10 – Loop credentials : Based on preset cases, each robot user is run through, checking for email in their mailbox. If relevant, 20.10 is run subsequently.
20.10 – Loop emails : Has a built-in Switch, where there must be one Case per XFlow form.
20.20 – Find attachments: Finds PDF and JSON files from email attachments.
20.30 – End processing : Counts +1 on SQL, moves the email to the processed folder in the mailbox, and resets all relevant variables so they do not interfere with the next loop.
30.10 – eDoc search: Used to search for a case, document or project. Return either an EdocCaseFileIdentifier or EdocProjektID .
EdocSearchFor | Possible variables for search |
Case | EdocCaseFileIdentifier EdocCase handler EdocCase number EdocType VarCPR |
Project | EdocProjectID EdocProjectNo. |
Document | EdocCaseFileIdentifier EdocDocumentID EdocDocumentNr EdocCase handler EdocType |
Default | ErrorList – ” 30.10 – Case type not recognized, skipped – %EdocOpretSag% ” Next Loop |
Error Handling | ErrorList – “30.10 – ScriptError occurred while searching – %ScriptError% ” CurrentMail is moved to ErrorMailFolder Next Loop |
Return | JSONEdocSearch EdocProjectID or EdocCaseFileIdentifier |
30.20 – eDoc create: Can be used to create the following
EdocCreateCase | Possible variables to create |
Citizen ( Citizen Template ) | EdocCategory EdocProjektID EdocCase handler EdocTemplate EdocTitle VarCPR |
Property ( Property Template ) | EdocCategory EdocProjektID EdocCase handler EdocTemplate EdocTitle VarBFE |
Case template | EdocCategory EdocProjektID EdocCase handler EdocTitle EdocSkabelon |
Case | EdocCategory EdocProjektID EdocCase handler EdocTitle EdocType |
Project | EdocCategory EdocCase handler EdocTitle |
Default | ErrorList – ” 30.20 – Case type not recognized, skipped – %EdocOpretSag% ” Next Loop |
Error Handling | ErrorList – ” 30.20 – ScriptError occurred while creating – ” %ScriptError% “ ” CurrentMail is being moved to ErrorMailFolder Next Loop |
Return | JSONEdocCreate EdocProjectID or EdocCaseFileIdentifier |
20.30 – eDoc journalize files: Journal the files associated with %ListUpload% . Please note that the document status is always set to “Final” , if you need something different, see the maintenance section and create a variable for this.
Overview | Variables |
Journalize | EdocCaseFileIdentifier EdocType EdocCase Handler EdocTitel ListUpload |
Error Handling | ErrorList – ” 30.30 – ScriptError occurred when journaling %EdocTitel% to %EdocSagsnummer% – ” %ScriptError% “ ” CurrentMail is moved to ErrorMailFolder Next Loop |
Return | JSONEdocJournalize EdocDocumentID |
40.00 – Example: Example of how to fill out a flow for a form. You can copy this and use it as a starting point for a new journal entry.
2.3 New blank checklist #
In 20.10 – Loop , a new case is created, which should be named the same as the email subject -PVA. The XFLowPDFName variable is filled with the file name of the PDF file with the XFlow form -pdf (the robot figures out (1) (2) and similar additional auto-numbering). If there are additional attachments to be journalized, BoolMultiAttachments is set to true. The PDF of the XFlow form will always be the main document, JSON is skipped as it is not relevant, and all other attachments are added to the list arbitrarily. If it is simple journalization, you can just fill in the variables for the journalization, and then run 30.30 – Edoc journalize files . If you need to extract data from the form, create a larger amount of code, then create a 40.xx +1 from the last created one, and fill this with the workflow. See 40.00 as an example. This is to avoid 20.10 becoming unmanageable.
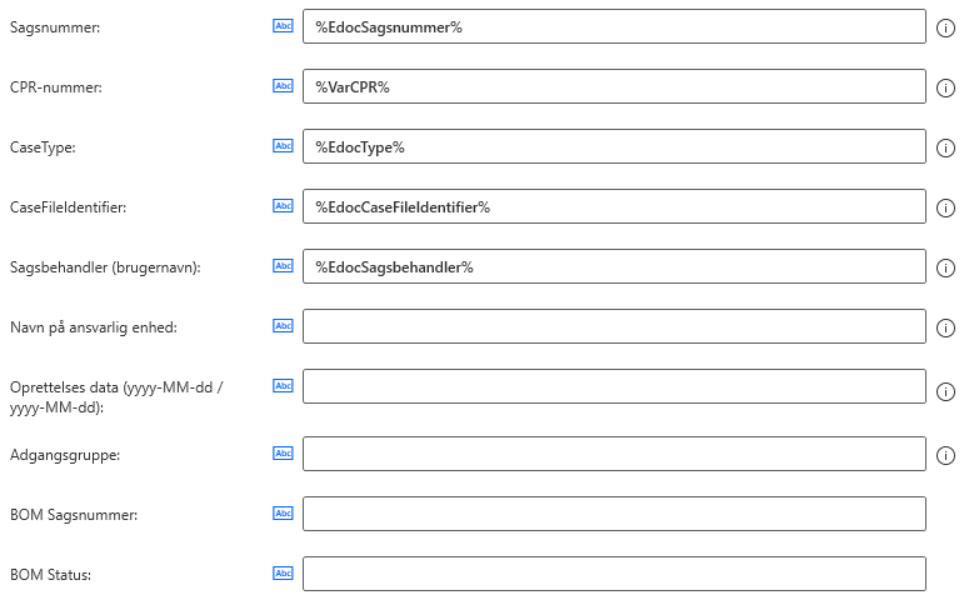
If you need to use more variables than those that exist, for example for logic, you are welcome to create an abbreviation and put it in front of the names, so it is easy to see which variables belong to which form. For example, if the form is called ” Holiday – Agreement on advance payment ” you can shorten it to, for example, HolidayAAF< variable name> and put the real variable name behind it. You can also put it in a comment at the top of the case.
2.4 Maintenance #
The most common maintenance will be the need to be able to fill in a variable for an API call that has not already been entered. It is directly ILLEGAL to hardcode it into the field, as it will affect all other forms in the future as well. If, as in the example below, you need BOM Case Number, it is legal to create a variable for this. However, it must be called Edoc + what is stated in the text, in the example EdocBOMSagsnummer . After that, you are welcome to check 30.10, 30.20 and 30.30 to see if other API calls also have this and fill it in.
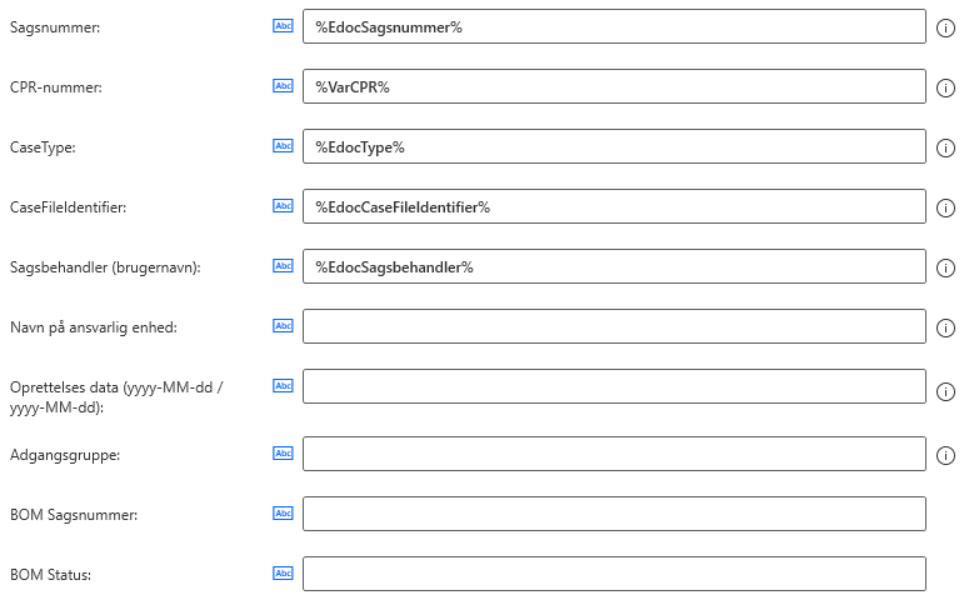
Finally – it MUST – be reset at 20.30 – End treatment in the region until reset. Or you risk it continuing to live on in the next loop.
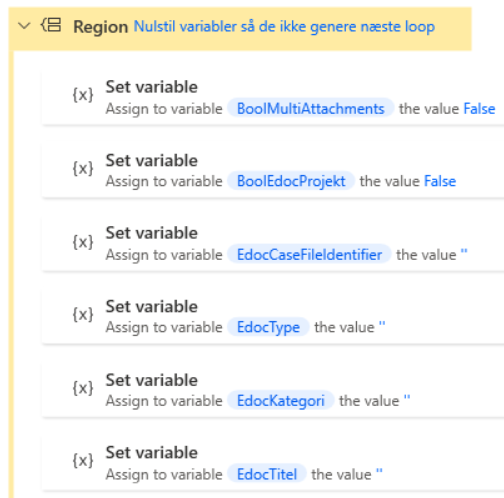