1. Introduction #
Selectors are what used to interact with User Interfaces (UI) or web pages. They are used to point to content within them, such as buttons or text fields, so they can be clicked.
Name | Note | Link |
jQuery Selectors | Overview of jQuery selectors | https://www.w3schools.com/jquery/jquery_ref_selectors.asp |
Regular Expressions | Section on regex with more sources for this | Regular Expressions (Regex) – RPA Help |
The easiest way to test selectors is to focus on the window, and just send the click, see if the robot catches it, and then tinker with the selectors again if it still doesn’t work. The shorter and more precise a selector is, the less maintenance you need to do in the future.

1.1 Example #
There are many ways to create selectors , and to make them as robust as possible, there are some standard solutions you can use. Typically, you can adjust your selectors via your RPA software. Below is an example, on Windows Calculator , where we need to press the 9 button.
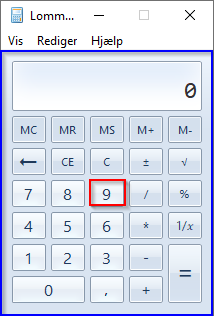
The selector for the 9-button might look like this. Note that there can be several levels in a selector , where we find parts behind it that help point to what we are looking for. In this case, the button sits on a pane , marked in blue in the image above, along with the remaining buttons. In this case, you can point to the button via its class and name , which should be unique enough to hit it. Alternatively, you can hit its id instead, marked in blue below. There will always be a bit of trial-and-error in this, to find out what a selector should contain in order to work. The less you need, the easier it is to maintain. At the same time, you may need to make it longer, to specify exactly what you are looking for.
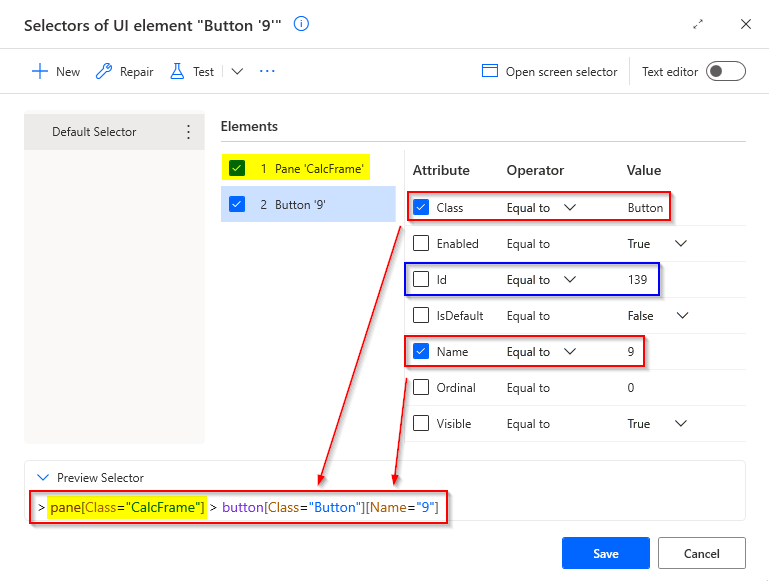
2. General #
2.1 Custom Selector #
In some cases, you can also build a custom selector that can dynamically choose between different elements. This can be an easier solution for selectors that are similar, but where the only difference is the name, for example. In our calculator example, we will have many selectors to maintain if we want one per button. Alternatively, we can create a dynamic selector that can be used instead.
Based on Power Automate Desktop , we can enable Text editor in the selector for our 9 button, and instead of 9 in Name , insert a variable.
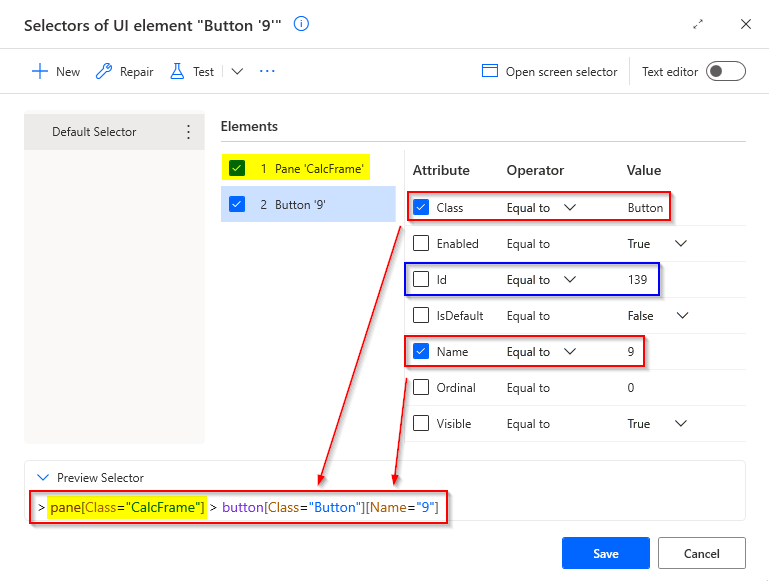
Later in the code, you can change the variable before sending a press, depending on which button you want to press.
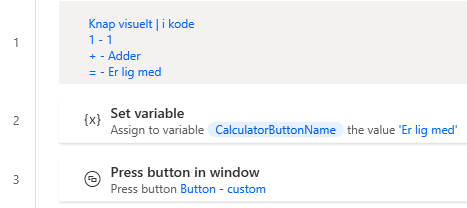
2.2 Regular Expressions (regex) match #
You can also use regular expressions (regex) in your controls to better match your selectors . For example, if there are IDs/names of a control that are partially autogenerated, these can be difficult to capture without regex .
In this example, we have an iframe that changes ID every time a new session is opened. So we don’t know what the numbers are going to be each time, but we know there will always be a variation of numbers. So we match directly on what we know, and use a (\d+) regex to say +x number of numbers that come here. The regex doesn’t care about the amount of numbers or what it is, it just finds something that matches the string that is inserted.
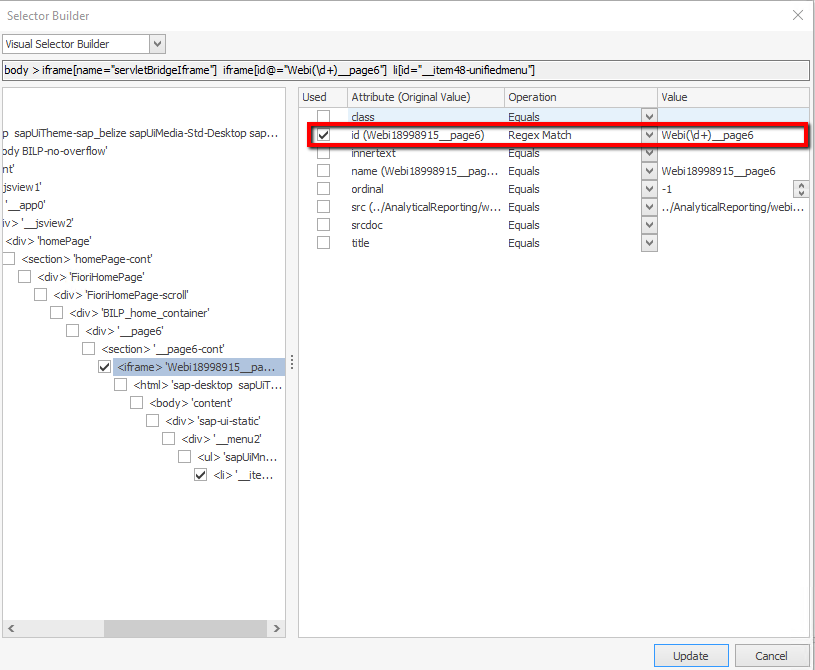
2.3 Find image #
Some RPA programs, including Power Automate Desktop , have the ability to find images on the screen, move the mouse, and optionally press. You take a picture of the button you are looking for ( similar to prtscrn in Snagit and Greenshot ), and then the robot tries to find the image on the screen itself. This can be used in both UI and Web automation.
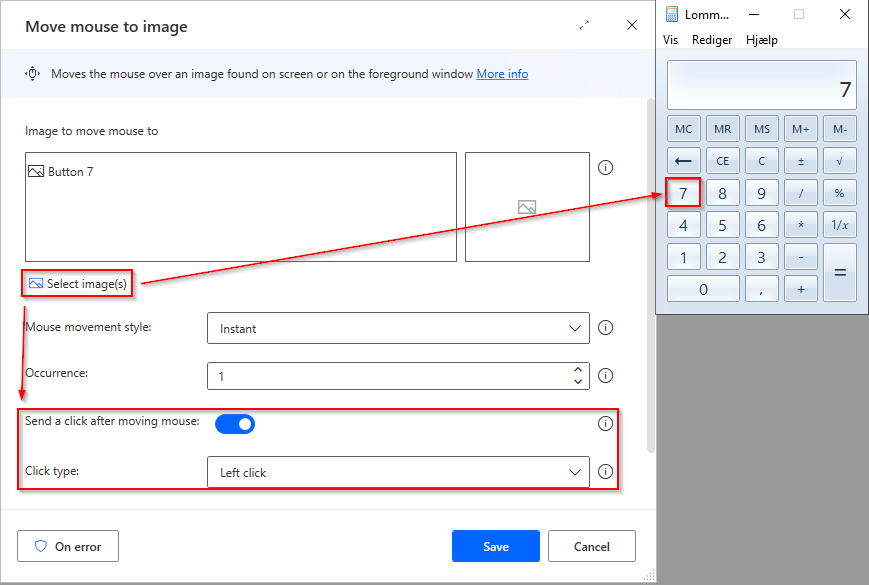
2.4 Validate input #
In some cases, you may need to extract a result or validate that an input you have made has now also been made. These can be filters you put in an application to sort data before extracting it, for example. Typically, this is used for console applications, where we do not otherwise really know what happens when we send clicks.
This example builds on the calculator example. There are two text fields, one keeping track of what has been typed, and the other of the current result / most recently inputted number.
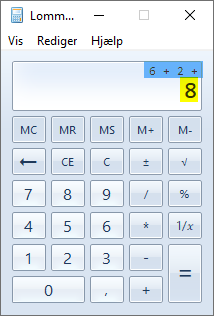
For example, in Power Automate Desktop , you have the option to extract the text from an attribute in the selector . From here, you can either extract the result from the calculator, marked in yellow above in the image, and use it in your code going forward. You can also extract the intermediate calculation, marked in blue in the image above, to validate that you have pressed the right things.
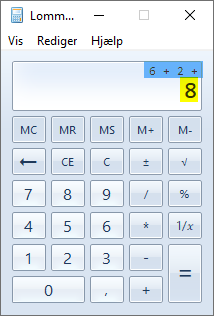
3. Web Automation – jQuery Selectors #
In Web Automation we have the additional option of using jQuery selectors , which can help to dynamically find selectors on websites. Typically these are used if a website has a lot of autogenerated code that does not have any unique or meaningful selectors to use. To use these, you need to create custom selectors .
For example, you can use a :contains(“Text”) behind your selector . This makes the selector look for a button that has a string pasted on it, with the text that has been typed in. Below is an example from msn.com where the selection can be changed to all the headings in the selected red field.
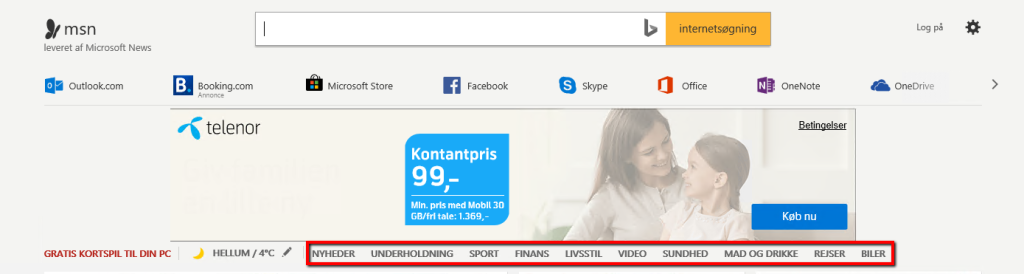
Before adjusting the code, where we hit via the li (list item) part of the selector
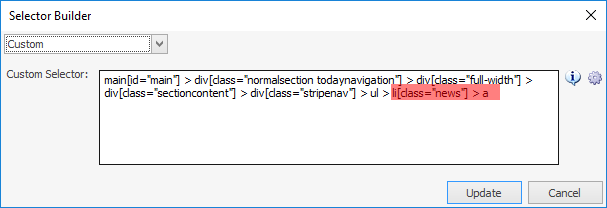
After adjusting the code where we hit the a (link) part of the selector
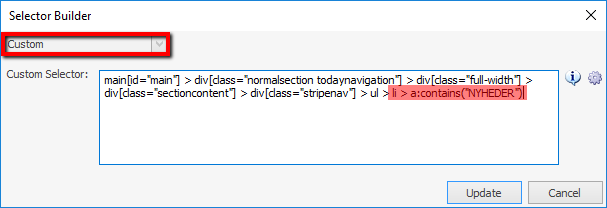